It is very useful to have a physics engine such as Box2D running if you want your game to be very interactive and mega awesome, but physics can be a bit of a pain to implement in your engine or game!
Lucky ours that we Box2D library (http://www.box2d.org/) provides a debug drawing interface!
What is that? When the world function "Step" is called, the physic calculations will happen, and the physic shapes will be updated with new positions, rotations and such.
After this positions are ready, you can make a call to DebugDraw so everything is renderered in the screen without worrying about game sprites or anything!
Hows does it work exactly? Based on virtual calls, of course!
The physics world object has a pointer to a b2DebugDraw object, and calls his virtual functions when it wants to draw something in the screen!
What is a virtual function? Well, its a function declared with the prefix "virtual" inside a class. This function can be called normally and even do some code..
The magic happens when you derive your own class from b2DebugDraw and override those virtual methods just by declaring and defining functions with the same name! You can define them with rendering code, OpenGL , for instance!
Then, you can tell Box2D to use your DebugDraw instead of the native one, and voila, everything appears on the screen, because Box2D will call the functions internally!
You can look at a example code here:
Box2D and Debug Drawing Explained
Enjoy boxing : )
Saturday, December 11, 2010
Friday, December 10, 2010
Pretty much Artistic (or not)
This post contains some "art" i do while i am still in the lowest bounds of my learning curve :P
VitalitySchedule Logo - High School Final Project

VitalitySchedule Logo - High School Final Project

Kurosaki Ichigo - Main Character from Bleach Anime show.
Drawing by hand, painted in photoshop!

NanoTech logo, NanoTech was a web-based game i've written in PHP for a school class.
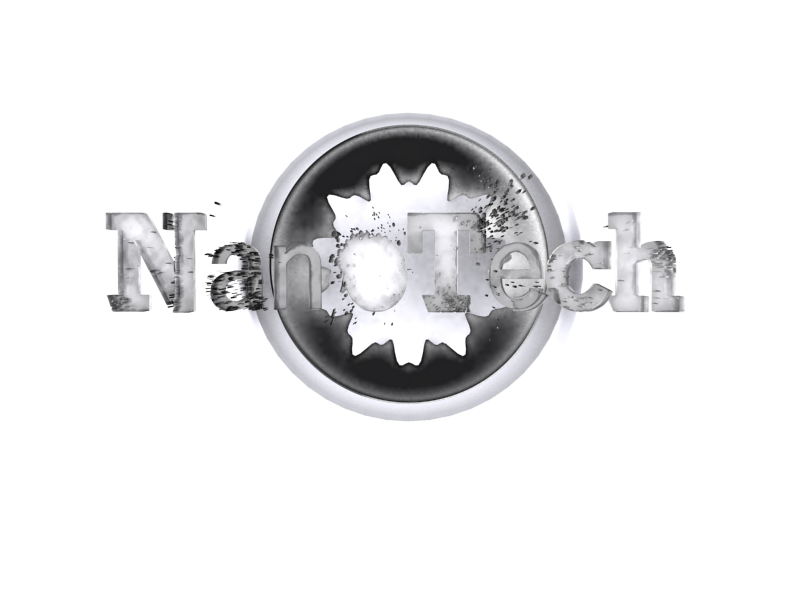
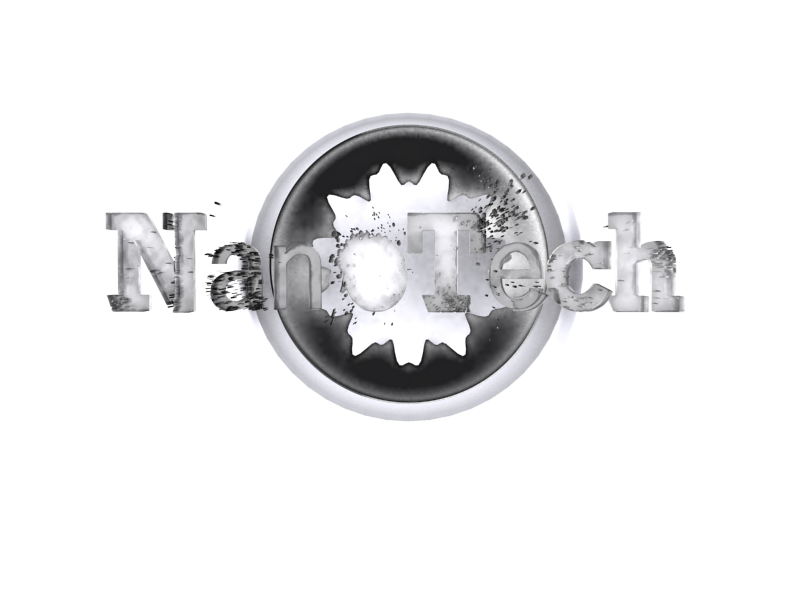
Tuesday, December 7, 2010
SlideParty - A fun logic game sideproject!
I am working on a fun sideproject which is a logic game, meant for all ages.
The gameplay isn't new to the world, but i really liked the idea, so i decided to make one for myself : )
The gameplay isn't new to the world, but i really liked the idea, so i decided to make one for myself : )
From the screenshot, you can have an idea of what the game is about, the blocks must be moved with the mouse, until a "master" piece is able to pass a "goal" in the bounds of the grid. It's pure logic, and easy to play. Will be posting more screenshots soon enough !
Monday, December 6, 2010
"Grudge" First Female Character
I've decided to use mostly pixel art to compose my game visuals. Though, i am going to build the characters in a 3D package, so i can have a lot of animations in a simpler and smoother way, in my opinion, and export the renders as bitmap animations. So, i will have my 2D art for characters being a "simulated" 3D and i will still have the 3D models to use in trailers, next games, whatever : )
I am using polygonal modeling to create the rough shape, then i add a smooth to get a better result. Sculpting will be ahaed : )
Here are two screenshots of the first night of work in the first female model, enjoy
I am using polygonal modeling to create the rough shape, then i add a smooth to get a better result. Sculpting will be ahaed : )
Here are two screenshots of the first night of work in the first female model, enjoy
More progress coming soon!
HollowEngine - Some progress
I've been working in the engine for a few weeks now, even tough i don't have much time, i try to implement some features sometime : )
The screenshot above is just a Testing Application screenshot from which you can see a couple of features i've been doing lately..
At left, you can see our charismatic friend homer simpson, which is actually running in the application, testing the animation system for sprites i've done. It allows the programmer to create the Animations in just a few lines of code, directly from a spritesheet.
In the middle, there is a random paint bitmap character. The fun thing about it is the circle cursor around him, which follows the mouse direction, always at the same distance relative to the character. Just some trigonometry. :)
Finnaly, you can see some game user interface. It is a librocket demo interface along with the debugger working!
More soon! :P
The screenshot above is just a Testing Application screenshot from which you can see a couple of features i've been doing lately..
At left, you can see our charismatic friend homer simpson, which is actually running in the application, testing the animation system for sprites i've done. It allows the programmer to create the Animations in just a few lines of code, directly from a spritesheet.
In the middle, there is a random paint bitmap character. The fun thing about it is the circle cursor around him, which follows the mouse direction, always at the same distance relative to the character. Just some trigonometry. :)
Finnaly, you can see some game user interface. It is a librocket demo interface along with the debugger working!
More soon! :P
Qt4 TV-Like Sleep Application for Windows PC
Hello, i've made a application in Qt4 that provides a easy interface to sleep your computer, a lot like TV sleep function.
The application itself is cross-platform, but the commands to temporize the shutdown/restart are windows-specific.
Its really, really simple, here i share the source code, and a binary version : )
Download:
SleepTime Binaries
SleepTime Source
Relevant Functions ( Dialog.cpp)
The application itself is cross-platform, but the commands to temporize the shutdown/restart are windows-specific.
Its really, really simple, here i share the source code, and a binary version : )
Download:
SleepTime Binaries
SleepTime Source
Relevant Functions ( Dialog.cpp)
void Dialog::on_pushButton_clicked()
{
//shutdown
QString time = QString::number(ui->lineEdit->text().toInt()*60);
QString command = "shutdown -s -t " + time;
system(command.toStdString().c_str());
}
void Dialog::on_pushButton_2_clicked()
{
//restart
QString time = QString::number(ui->lineEdit->text().toInt()*60);
QString command = "shutdown -r -t " + time;
system(command.toStdString().c_str());
}
void Dialog::on_pushButton_3_clicked()
{
//cancel
system("shutdown -a");
}
Sunday, December 5, 2010
Installing Qt4 with MySQL plugin (QMYSQL) in Windows (gcc)
Note: If you feel like you already tried everything, check the last highlighted paragraph, may be useful : )
I needed Qt4 with MySQL support under windows to develop my high school final project. The thing is i had some trouble setting it up, but i found a way eventually : )
The same way it worked for me, i hope it works for you too! ^^
So, to begin download a qt sdk package: http://qt.nokia.com/downloads
Install it somewhere and make sure it is ready to use without the mysql support.
Open the Qt Command Prompt, that comes within the SDK installation, it will be used for getting the mysql library compatible with mingw32, then feeding qt the needed files, and finnaly recompile the package.
Next thing in the list, download the mysql library: http://www.mysql.com/downloads/mysql/ install it in somewhere easy to go in the console. Make sure you install the header files, in the installation options.
Download the mingw-utils: https://olex.openlogic.com/packages/mingw-utils You will need a tool contained in it.
Take the reimp tool from the mingw-utils package, and place it in mingw bin folder, same place you will see a dlltool executable. This should be in the Qt installation, in a mingw subfolder, look for it : )
In the console, go to the mysql library folder you installed a bit ago. Then change directory to /lib/opt and run the following commands as shown in: http://www.rag.com.au/linux/qt4howto.html
c:\mysql\lib\opt> reimp -d libmysql.lib
c:\mysql\lib\opt> dlltool --input-def libmysql.def --dllname libmysql.dll --output-lib libmysql.a -k
By now, you have everything Qt4 needs to build MySQL support but Qt does not know where to find it yet. Just move that libmysql.a file you generated with the previous command and put it in the mingw directory, under the lib subdirectory. Then copy all the files in %mysqlinstall%/include to the mingw directory, under include subdirectory. The libraries are all set up for qt installation.
Now just do the traditional configure step to rebuild. In the console, go to qt default directory, where configure.exe is.
Run:
configure -platform win32-g++ -qt-sql-mysql (Note: you can add whatever options you would like, to complement your rebuild, your choice)
mingw32-make
This will take a lot of time, probably more than 2 hours. Be patient : )
While you wait, there is one more thing to solve. When i did all this, my Qt4 was still not able to load the QMYSQL plugin, and since everything was installed correctly, it was a runtime issue. In theory, it would run just fine by adding the libmysql.dll to the qt bin directory, or to the application directory. but this wouldn't help.
[ Luckily, i found the way around with the dependency walker. The application was requesting libmysql.a instead of libmysql.dll, so the final step:
Copy from the mysql installation, in the bin directory, the libmysql.dll, paste it into the qt bin directory, where you will find a lot of other dll files. After this, rename the libmysql.dll to libmysql.a.]
After all these steps, your Qt4 should be working as charm. Hope it helps you. Have fun ! : )
I needed Qt4 with MySQL support under windows to develop my high school final project. The thing is i had some trouble setting it up, but i found a way eventually : )
The same way it worked for me, i hope it works for you too! ^^
So, to begin download a qt sdk package: http://qt.nokia.com/downloads
Install it somewhere and make sure it is ready to use without the mysql support.
Open the Qt Command Prompt, that comes within the SDK installation, it will be used for getting the mysql library compatible with mingw32, then feeding qt the needed files, and finnaly recompile the package.
Next thing in the list, download the mysql library: http://www.mysql.com/downloads/mysql/ install it in somewhere easy to go in the console. Make sure you install the header files, in the installation options.
Download the mingw-utils: https://olex.openlogic.com/packages/mingw-utils You will need a tool contained in it.
Take the reimp tool from the mingw-utils package, and place it in mingw bin folder, same place you will see a dlltool executable. This should be in the Qt installation, in a mingw subfolder, look for it : )
In the console, go to the mysql library folder you installed a bit ago. Then change directory to /lib/opt and run the following commands as shown in: http://www.rag.com.au/linux/qt4howto.html
c:\mysql\lib\opt> reimp -d libmysql.lib
c:\mysql\lib\opt> dlltool --input-def libmysql.def --dllname libmysql.dll --output-lib libmysql.a -k
By now, you have everything Qt4 needs to build MySQL support but Qt does not know where to find it yet. Just move that libmysql.a file you generated with the previous command and put it in the mingw directory, under the lib subdirectory. Then copy all the files in %mysqlinstall%/include to the mingw directory, under include subdirectory. The libraries are all set up for qt installation.
Now just do the traditional configure step to rebuild. In the console, go to qt default directory, where configure.exe is.
Run:
configure -platform win32-g++ -qt-sql-mysql (Note: you can add whatever options you would like, to complement your rebuild, your choice)
mingw32-make
This will take a lot of time, probably more than 2 hours. Be patient : )
While you wait, there is one more thing to solve. When i did all this, my Qt4 was still not able to load the QMYSQL plugin, and since everything was installed correctly, it was a runtime issue. In theory, it would run just fine by adding the libmysql.dll to the qt bin directory, or to the application directory. but this wouldn't help.
[ Luckily, i found the way around with the dependency walker. The application was requesting libmysql.a instead of libmysql.dll, so the final step:
Copy from the mysql installation, in the bin directory, the libmysql.dll, paste it into the qt bin directory, where you will find a lot of other dll files. After this, rename the libmysql.dll to libmysql.a.]
After all these steps, your Qt4 should be working as charm. Hope it helps you. Have fun ! : )
High School Project - VitalitySchedule
As my 3-year programming course was ending, it was requested to create a software solution applicable in real world, using databases and such.
I chose to create a software that would serve a clinic of variable size, by creating a system to provide the basic functions needed to run such a business.
I wanted the system to be adaptative and every peer to be connected in a network, so, i programmed my software in a client-server basis.
The server is the only one who has access to the MySQL database, holding all important data. The server application, will hold a client per thread and provide each one all functionality needed. A client can be a clerk worker, serving the patients and scheduling them for specific hours, a doctor or a system administrator.
The client starts on a login interface, that upon authentication, decides which interface to show. Based on this, each user will get different access acording to its access level.
To create this software, i used Qt4 Framework with support for MySQL databases. Development time was around 2 weeks.
There is not a lot more to say, i rather show some screenshots of its features and release the code as opensource under LGPL license, just as Qt Framework . :)
You can download all the source from here: https://sourceforge.net/projects/vitalityschedul/files/
In the archive you will find the server module (core), client module (user) and a Database Model, which will require MySQL Workbench to use.
Screenshots:
1 - Login Panel
2 - Patient Browsing and Editing Panel
3 - Scheduling Panel
(That is not all features)
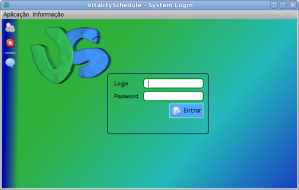
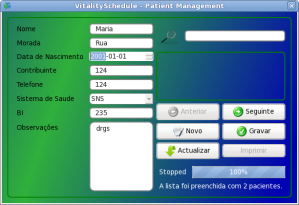
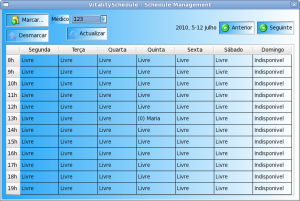
Enjoy
I chose to create a software that would serve a clinic of variable size, by creating a system to provide the basic functions needed to run such a business.
I wanted the system to be adaptative and every peer to be connected in a network, so, i programmed my software in a client-server basis.
The server is the only one who has access to the MySQL database, holding all important data. The server application, will hold a client per thread and provide each one all functionality needed. A client can be a clerk worker, serving the patients and scheduling them for specific hours, a doctor or a system administrator.
The client starts on a login interface, that upon authentication, decides which interface to show. Based on this, each user will get different access acording to its access level.
To create this software, i used Qt4 Framework with support for MySQL databases. Development time was around 2 weeks.
There is not a lot more to say, i rather show some screenshots of its features and release the code as opensource under LGPL license, just as Qt Framework . :)
You can download all the source from here: https://sourceforge.net/projects/vitalityschedul/files/
In the archive you will find the server module (core), client module (user) and a Database Model, which will require MySQL Workbench to use.
Screenshots:
1 - Login Panel
2 - Patient Browsing and Editing Panel
3 - Scheduling Panel
(That is not all features)
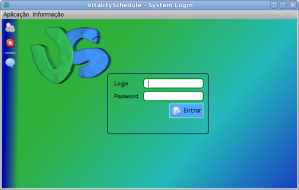
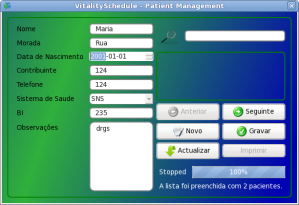
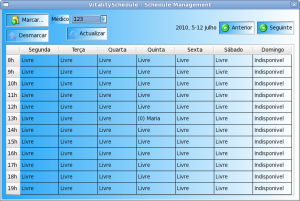
Enjoy
HollowEngine-based Project - Codename Grudge
Hey, i am starting a new project i've been conjuring in my mind the last weeks, it is a platformer 2D game with a fight-oriented gameplay. It is being developed using the hollowengine to its most. I dont have a definitive name for it yet and so i simply called it Grudge.
It is meant to be published in Steam as a "Indie Game", more or less. It is one of my first projects aiming for a commercial use, so it won't be expensive : )
About the plot, its not defined yet, but i do have some ideas i would like to bring to life. There would be a religious concept at its core, but also "proofs" that religion is something different than we think, like the skills the characters can do being explained by "humanly" abilities. The world is set in modernity, except there was a cataclysm of some kind, and technology is gone. No more guns. There will be some selected people who have abilities that others don't, leading them to fight all the evil, as usually. The same old drill, with a entirely new concept and gameplay.
I will be posting more when progress is made. Stay tuned.
It is meant to be published in Steam as a "Indie Game", more or less. It is one of my first projects aiming for a commercial use, so it won't be expensive : )
About the plot, its not defined yet, but i do have some ideas i would like to bring to life. There would be a religious concept at its core, but also "proofs" that religion is something different than we think, like the skills the characters can do being explained by "humanly" abilities. The world is set in modernity, except there was a cataclysm of some kind, and technology is gone. No more guns. There will be some selected people who have abilities that others don't, leading them to fight all the evil, as usually. The same old drill, with a entirely new concept and gameplay.
I will be posting more when progress is made. Stay tuned.
Wednesday, December 1, 2010
HollowEngine
Good morning world!
I am presenting the concept idea and some of the up-to-date work on the HollowEngine i am working on.
This Engine is meant to create games, as you may have tought, obviously ! The engine will have its primary focus in platforming games, but will also support top-down games, along with logic games.
In sum, its a 2D engine to build casual games if not something commercial.
Knowing i am no superhero(yet!) i decided to create the engine on top of a few libraries!
So,
-SFML is used for graphics, input, sound
-Box2D provides the physics layer
-libRocket provides the interface systems
-SPARK particles provide the cool effect stuff
-Enet provides the networking capabilities
I am using c++ object oriented design to build this, as you may have noticed : )
This engine creations won't try to mimic old classic games, instead, it will try to give the best of the modern approaches to the users. It will be physicly oriented all the time. One of the main issues will be creating networked simulations with it because playing alone is long gone in time :)
I am coding a world editor tool, where you can create the maps to use with the engine very easily.
The engine itself is going to be cross-platform , so will the games. About this tool, for now its built on top of .NET, but in future maybe it will have a twin in another platform.
Screenshot of the editor:
I am presenting the concept idea and some of the up-to-date work on the HollowEngine i am working on.
This Engine is meant to create games, as you may have tought, obviously ! The engine will have its primary focus in platforming games, but will also support top-down games, along with logic games.
In sum, its a 2D engine to build casual games if not something commercial.
Knowing i am no superhero(yet!) i decided to create the engine on top of a few libraries!
So,
-SFML is used for graphics, input, sound
-Box2D provides the physics layer
-libRocket provides the interface systems
-SPARK particles provide the cool effect stuff
-Enet provides the networking capabilities
I am using c++ object oriented design to build this, as you may have noticed : )
This engine creations won't try to mimic old classic games, instead, it will try to give the best of the modern approaches to the users. It will be physicly oriented all the time. One of the main issues will be creating networked simulations with it because playing alone is long gone in time :)
I am coding a world editor tool, where you can create the maps to use with the engine very easily.
The engine itself is going to be cross-platform , so will the games. About this tool, for now its built on top of .NET, but in future maybe it will have a twin in another platform.
Screenshot of the editor:
More news coming soon along with screenshots : )
Blog fresh start !
Hello everyone, i'm happy to say i will continue my blogging experience in blogspot :)
This blog is going to continue the work in codetimestories.wordpress.com, a lot of new stuff will appear soon.
Stay tuned ; )
This blog is going to continue the work in codetimestories.wordpress.com, a lot of new stuff will appear soon.
Stay tuned ; )
Subscribe to:
Posts (Atom)